With the rise of fintech and digital trading, accessing financial data has become easier than ever before. For investors and traders who rely on timely and accurate market data for analysis and decision making, leveraging investing APIs in Python provides a convenient and efficient way to streamline the data collection process. In this comprehensive guide, we will explore some of the most popular investing APIs for Python and how to use them to extract real-time and historical data on stocks, ETFs, funds, forex, cryptocurrencies, and more. Whether you are looking to develop algorithmic trading systems or conduct quantitative research, these Python APIs offer robust capabilities to acquire a rich set of investment data from global exchanges and platforms.
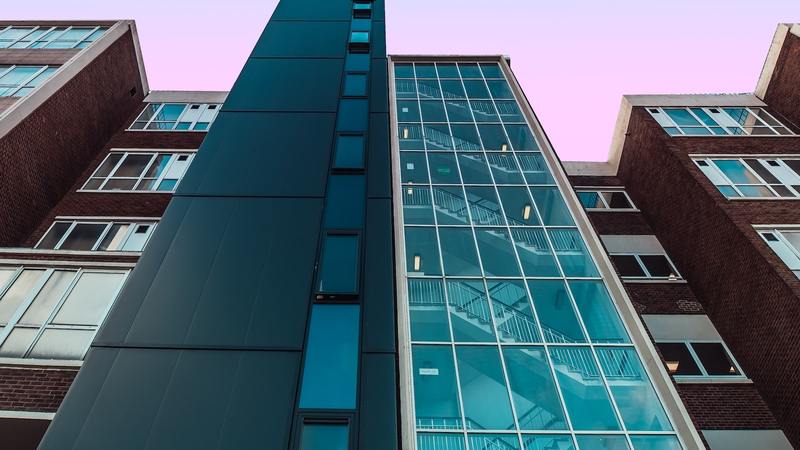
Overview of Major Investing APIs Compatible with Python
Here is a rundown of some of the top investing APIs that provide Python support and access to a wide range of securities data across global markets:
Yahoo Finance API – Offers free access to real-time and historical prices, fundamentals data, market caps, EPS, dividends, news, and more across stocks, ETFs, mutual funds, currencies, and cryptocurrencies. Discontinued in 2017 but still accessible through unofficial Python wrappers.
Tiingo API – Provides clean, reliable, and affordable market data. Covers equities, ETFs, mutual funds, FOREX, and cryptocurrencies. Daily historical data available up to 15+ years. Python SDK available.
Polygon API – Low latency, high quality market data API that offers real-time and historical equities and futures data, forex data, developer APIs, and more. Python SDK available.
IEX Cloud API – API service from IEX stock exchange that offers historical and intraday data on stocks, ETFs, mutual funds, FOREX, Cryptocurrencies, commodities, bonds, rates, economic data, and news. Python SDK available.
Twelve Data API – Global market data API covering real-time quotes, historical data, fundamentals, earnings estimates, economic data, commodities, ETFs, forex, indices, cryptocurrencies. Python SDK available.
Alpha Vantage API – Global equity, forex, cryptocurrency data. Real-time and historical equities data from NYSE, NASDAQ, London Stock Exchange etc. Python SDK available.
Using these industry-leading investing APIs along with Python’s data analysis capabilities provides tremendous potential to build custom financial analysis tools, backtest trading strategies, automate trade execution, and more.
Key Features and Data Offerings of Investing APIs
While offerings differ across providers, most major investing APIs offer the following essential features and data streams useful for investment analysis and trading:
– Real-time streaming quotes for stocks, ETFs, funds, forex, cryptocurrencies
– Intraday minute-level and daily OHLC pricing data
– Adjusted close prices and dividends data
– Comprehensive fundamentals data – financials, valuation ratios, earnings, dividends
– Company profiles, business description, corporate actions
– Analyst estimates, ratings, target prices
– News, press releases, earnings call transcripts, SEC filings
– Sector/Industry classification for equities
– Market trading hours, holidays, exchange calendars
– Global coverage across major asset classes and exchanges
By leveraging these data capabilities through Python, investors can develop financial models, build trading algorithms, backtest investment strategies, analyze securities using technical and fundamental indicators, and ultimately make more informed investment decisions.
Step-by-Step Tutorials to Access Investing APIs with Python
Most investing APIs provide detailed documentation along with Python SDKs or wrappers to make it easy to get started. Here are some step-by-step tutorials to access key investing data using sample Python code:
**Getting Real-Time Stock Quotes**
“`python
# Import Twelve Data API Python module
from twelve_data_sdk import TDClient
# Initialize API client with key
td = TDClient(api_key=’YOUR_API_KEY’)
# Get real-time quote for Apple stock
quote = td.get_quote(‘AAPL’)
print(quote[‘open’])
print(quote[‘high’])
“`
**Fetching Historical Price Data**
“`python
# Import AlphaVantage API Python module
from alpha_vantage.timeseries import TimeSeries
# Initialize API client with key
ts = TimeSeries(key=’YOUR_API_KEY’)
# Get 2 years daily prices for Tesla stock
tesla_data, meta_data = ts.get_daily(symbol=’TSLA’, outputsize=’full’)
# Print date and open price
for date, daily_data in tesla_data.items():
print(date, daily_data[‘1. open’])
“`
**Screening Stocks by Fundamentals**
“`python
# Import IEX Cloud API Python module
import iexfinance as iex
# Initialize API client with key
iex_client = iex.Client(api_token=’YOUR_API_KEY’, version=’stable’)
# Screen for stocks with P/E ratio < 20 screen = iex_client.filter(symbol=['AAPL', 'MSFT', 'GOOG'], filter='peRatio<20') # Print matching stocks print(screen) ``` With some basic Python knowledge, investors can rapidly build out customized financial analysis tools and trading systems leveraging these easy-to-use investing APIs. Python offers a vast array of investing APIs to securely access real-time, intraday, and historical market data across global exchanges. Leading investing APIs from data providers like Polygon, Twelve Data, and IEX Cloud provide comprehensive coverage of equities, funds, forex, cryptocurrencies, company fundamentals, earnings, and more. By leveraging these APIs, investors can develop powerful programs for backtesting, algorithmic trading, quantitative modeling, and making data-driven investment decisions.